JSP 내장 객체인 세션을 이용해서 간단하게 장바구니를 구현했다.
Login.jsp : 사용자 이름을 입력
setProduct.jsp : 리스트에서 원하는 상품 추가
add.jsp : 선택한 상품을 세션에 넣는다. 다시 상품페이지로 이동
checkOut.jsp : 선택한 상품의 목록을 보여준다. (계산)
+
logout.jsp : 로그아웃 클릭시 세션 초기화 ( session.invalidate() )
clear.jsp : 장바구니 비우기를 구현하기 위해 추가함
📝 결과물
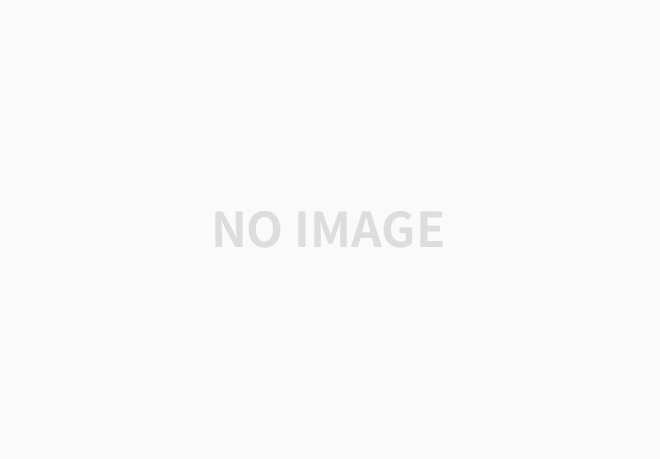
⦁ 1. Login.jsp
🧾 소스코드
더보기
<%@ page language="java" contentType="text/html; charset=EUC-KR"
pageEncoding="utf-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>로그인</title>
</head>
<body>
<%
//로그인이 되어있으면 제품페이지로 pass
if(session.getAttribute("login") != null){
response.sendRedirect("setProduct.jsp");
}
%>
<center>
<h3>로그인</h3>
<hr/>
<form action="setProduct.jsp">
이름 : <input type="text" name="name">
<input type="submit" value="로그인">
</form>
</center>
</body>
</html>
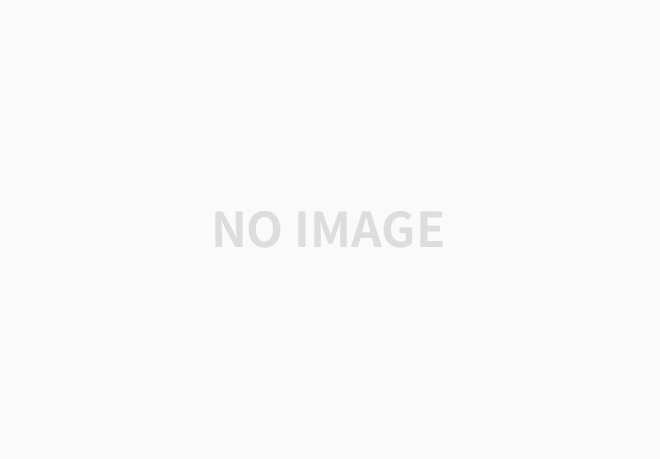
⦁ 2. setProduct.jsp
<%
request.setCharacterEncoding("utf-8");
String name = request.getParameter("name");
//이름이 ""이면 돌려보냄
if(name == ""){
out.println("<script>alert('이름을 입력해주세요.');</script>");
out.println("<script>location.href='Login.jsp'</script>");
}
//세션에 login이 없다면 등록
if(session.getAttribute("login") == null){
session.setAttribute("login", name);
}
%>
🧾 전체 소스코드
더보기
<%@ page language="java" contentType="text/html; charset=EUC-KR"
pageEncoding="utf-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>제품 페이지</title>
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
String name = request.getParameter("name");
//이름이 ""이면 돌려보냄
if(name == ""){
out.println("<script>alert('이름을 입력해주세요.');</script>");
out.println("<script>location.href='Login.jsp'</script>");
}
//세션에 login이 없다면 등록
if(session.getAttribute("login") == null){
session.setAttribute("login", name);
}
%>
<center>
<h3>제품 페이지</h3>
<hr/>
<%= session.getAttribute("login") %>님이 로그인 한 상태입니다. <br/>
<hr/>
<form action="add.jsp">
<select name="fruit">
<option>Apple</option>
<option>Kiwi</option>
<option>Mango</option>
<option>Pineapple</option>
</select>
<input type="submit" value="담기">
</form>
<br/>
<a href="checkOut.jsp">계산</a>
</center>
</body>
</html>
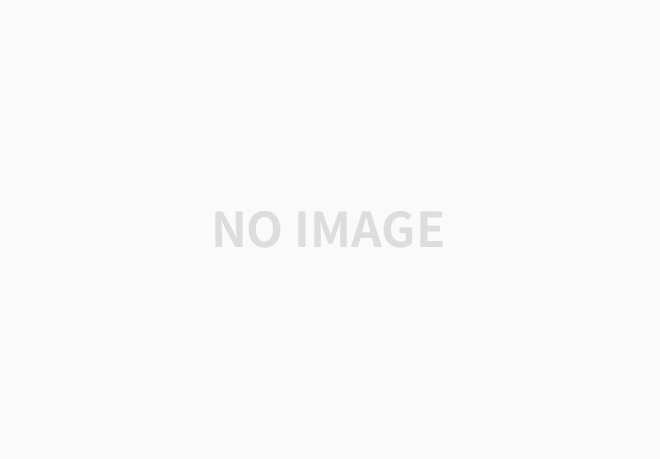
⦁ 3. add.jsp
세션에도 ArrayList를 저장할 수 있다.
❗ 주의점
session.getAttribute()로 데이터를 가져올 경우 Object 타입으로 리턴해주기
때문에 적절한 형변환이 필요하다.
ArrayList<String> arr = (ArrayList)session.getAttribute("fruitList");
🧾 소스코드
더보기
<%@ page language="java" contentType="text/html; charset=EUC-KR"
pageEncoding="utf-8"%>
<%@ page import="java.util.*"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>장바구니</title>
</head>
<body>
<%
ArrayList<String> arr = (ArrayList)session.getAttribute("fruitList");
//세션에 ArrayList 등록
if(arr == null){
arr = new ArrayList<String>();
session.setAttribute("fruitList", arr);
}
//파라미터 ArrayList에 추가
request.setCharacterEncoding("utf-8");
String fruit = request.getParameter("fruit");
arr.add(fruit);
//alert 띄우고 2페이지로 돌려보냄
out.println("<script>alert('저장되었습니다.');</script>");
out.println("<script>location.href='setProduct.jsp'</script>");
%>
</body>
</html>
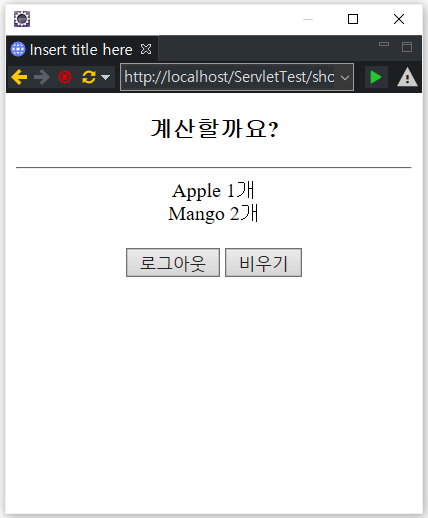
⦁ 4. checkOut.jsp
Collections.frequency(arr, "찾을 문자열")
메서드를 사용해서 ArrayList 안에 있는 찾을 원소의 개수를 구할 수 있다.
로그아웃 버튼을 누르면 session.invalidate() 메서드를 통해 세션을 초기화한다.
따라서, 로그아웃을 하지 않고 종료했다면 세션에 데이터가 남아있어서 바로 제품 페이지로 이동한다.
비우기 버튼을 누르면 clear.jsp 페이지로 간 후, 세션에 있는 과일 ArrayList를 null로 변경한다.
그 후, 다시 제품 페이지로 이동시킨다.
//logout.jsp 파일
<%
session.invalidate(); //세션 초기화 메서드
response.sendRedirect("Login.jsp");
%>
//clear.jsp 파일
<%
session.setAttribute("fruitList", null);
response.sendRedirect("setProduct.jsp");
%>
🧾 소스코드
더보기
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<%@ page import="java.util.*"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Insert title here</title>
</head>
<body>
<center>
<h3>계산할까요?</h3>
<hr/>
<%
ArrayList arr = (ArrayList) session.getAttribute("fruitList");
String[] category = {"Apple","Kiwi","Mango","Pineapple"};
if(arr != null){
for(int i=0; i<category.length; i++){
int count = Collections.frequency(arr, category[i]);
if(count >= 1) {
out.println(category[i] +" "+ count +"개<br>");
}
}
}
%>
<br/>
<button type="button" onclick="location.href='Login.jsp'">로그아웃</button>
<button type="button" onclick="location.href='clear.jsp'">비우기</button>
</center>
</body>
</html>
참고 문헌 :
'Front-End > JSP' 카테고리의 다른 글
[JSP] JSP에서 DB연동하기 (JDBC, 오라클, 커넥션 풀) (3) | 2022.10.08 |
---|---|
[JSP] 자바빈즈로 회원가입 페이지 구현하기 (useBean 액션 사용, 미사용 버전 2가지) (1) | 2022.09.21 |
[JSP] JSP 내장 객체 application 메서드 및 예제 (2) | 2022.09.19 |
[JSP/Servlet] 서블릿 구현하기 (HttpServlet, web.xml, 매핑이란?) (2) | 2022.09.16 |
[JSP] 서블릿(Servlet)과 JSP 정리, MVC 패턴 (8) | 2022.09.15 |